Intro
Let's start with some story time !
Once upon a time, there was a language called Java that was widely used by tech companies-and still- to develop web, desktop and mobile applications with high performance and scalability. For now, let's put our focus on mobile applications as the use of smarthphones became a necessity and especially for android applications because Android OS has the largest install base of any operating system; And it's not just for phones or tablets but also when it comes to smart TVs, cars and smart watches etc ... So that's why I was drawn to make this comparison out of curiosity.
Java has always been the only default programming language in Android until Google announced the new addition to its latest Android (Android Oreo) : a brand new programming language called Kotlin. Kotlin had been under developement for six years by JetBrains since 2011 and the first version v1.0 was released in 2016 after Google decided to partner with JetBrains development team to bring support to Android.
So the question to be raised will be:
Java or Kotlin, which is better for Android mobile applications?
In order to potentially find a final answer to this question, I decided to lead this comparison by invoking 3 different aspects: performance, language features and paradigms, so I basically focused on the developers experience more.
Language Features
Apparently, kotlin was created to try to address some issues that Java has. In this section, I will try to bring up the solutions solved in Kotlin that were once a pain for Android Developers. Here are some important features:
Feature | Explications |
---|---|
Interoperability | Java and Kotlin are interoperable which means we can find both type of files in the same project as well as they can invoke each others librairies. |
Data Classes | In Java, in order to write a POJO, you have to declare the fields, write the constructor and also the getters and the setters of all attributes, too much code isn't it? Well, in Kotlin, one line of code is fairly sufficient as shown in the example below: data class User(var name: String, var age: Int) |
Extension functions | In Java, if we want to add a new funtionnality to a class, we will have to inherit from this class or use the Decorator design pattern. So the goal in Kotlin is to not modify the source code of the class we want to extend by adding new members but rather create new functions that we can call using this class objects, those functions are called extension functions. |
Immutability | Like in Java, we use the keyword final if we want to declare a variable that won't be reassigned later. In Kotlin, there are the var and val keywords to manage the immutability of a property. So a property declared with var has a setter and a getter (mutable) while a property declared with val has a getter and a private setter (immutable) and the difference between final and val is that val is thread safe. |
Lambda expressions | Even the latest Android version supports only java 7 and lambda expressions were added until Java 8 so that's why Kotlin developers added lambdas support to Kotlin to resolve this problem. Lambda expressions are anonymous functions that can be passed in an argument of another function. |
Null safety | One of the common exceptions thrown in Java is the NullPointerException so Kotlin makes all types non-nullable by default so if a developer needs to use variables that hold a null value, it has to be declared as a null value like this : var toto: String? so if we want to access toto, the compiler will do a null check on the nullable variable. |
Type aliases | This feature improves the code readibility by assigning a name to a complex type.
|
Anko | I'm specifically interested in the Anko layouts which is a DSL that allows us to build UI's with zero XML code. For example, to create a fieldtext and a button with a listener with Kotlin, we can write the code below instead of creating the XML layout and then binding the view and the data in a Java class:
|
Now that we've actually seen some code features and functionnalities that were added in Kotlin we must however test the performances of the language when it comes to rapidity and its behaviour in different use cases and that's what we will represent in the next section.
Performances
In this section, I will try to mention the most remarquable aspects that would make an Android application that is based on Java different from the one based on Kotlin:
Code size:
According to previous features we can say that Kotlin requires much less lines of code so for example,a java application that has 5,491 methods and 12,371 lines of code was reduced to to 4,987 methods and 8,564 lines of code after converting it to Kotlin.
Build speed:
Before getting straight into analysis, I would like to clear up some build concepts and tools. Kotlin projects can be build by multiple build tools like Ant, Maven, Gradle ... Here I'll be interested in Gradle as it is the most common build tool used in android projects. There are multiple ways to build a project: Clean builds and incremental build.
what is the difference between these two?
Clean build compiles all source files in code whether they were changed or not but incremental build will compile only the modified files and the ones related to them. This difference approach can make a performance difference using these two types of compilation.
Next, I will be representing the results of building both Java in Kotlin code using clean and the incremental build and study the differences and similarities.
1. Clean builds
The two scenarios here are activating the Gradle daemon and then deactiviting it to spot if there are any differences. Usually when building a Java code, one will stop the JVM each time a build is done hence the performance that was once improved after multiple builds will be lost, the role of the Gradle daemon here is that it maintains the improved performance while staying alive between builds.
Joggling between these two scenarios can give us different results:
- Execute without Gradle daemon (worst case scenario) : Java compiles 17% faster than Kotlin.
- Execute while Gradle daemon activated : Java compiles 13% faster than Kotlin.
In the bar charts above, each couple of bars represents the time (in seconds) that each build has taken, we notice that there are 10 coupled bar charts it means ten consecutive builds were executed and after each build the duration was written down to finally capture the compilation speed rate difference between both languages. The reason why it's been tested out with multiple builds is that usually compilation takes multiple attempts to be improved.
Why is Java faster in clean build in both scenarios?
It is most likely that Kotlin needs a little more warmup than Java does, but is it genuine to compare the speed of two languages? I'll give more details after completing the next scenario.
2. Incremental builds
This second scenario consists of using the incremental build. Built-in tasks, like JavaCompile declare a set of inputs (Java source files) and a set of outputs (class files). Gradle uses this information to determine if a task is up-to-date and needs to perform any work. If none of the inputs or outputs have changed, Gradle can skip that task. That's what we call Gradle's incremental build
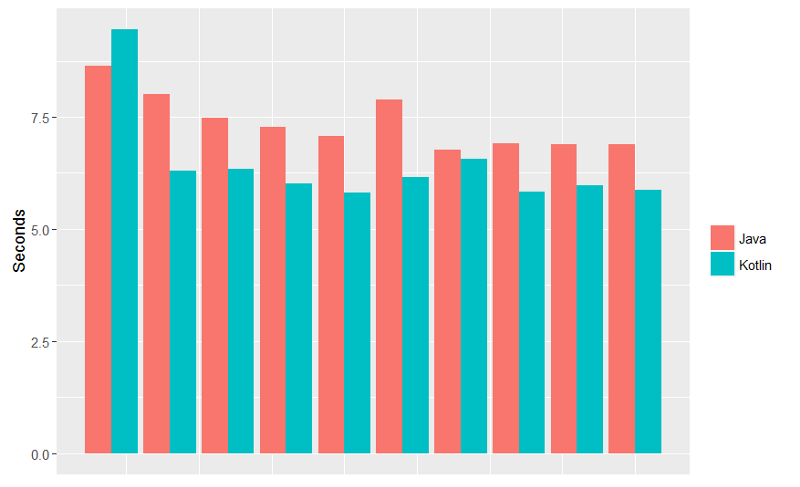
Same thing here, 10 consecutive builds has been tested out and we notice that in the first build Java is faster than Kotlin but after few builds they became almost the same so we can say that Kotlin performs better in incremental build which is basically the most common scenario in the developers' world.
Conclusion
The question of whether this language is faster than another is kind of meaningless in this case because the language performance depends on its runtime, the operating system and the code. While both Java and Kotlin are JVM languages which means their both compilers generate the same bytecode. Hence, even though in the previous scenarios seems that their performances are a little different, I still think there are no apparent reason for both languages to be so much different when it comes too build speed. It's rather up to the programmer to write an optimized and clean code and most important to take advantage of great build-in functionalities provided by a language
Test application:
I chose 3 common use cases to test an android app based on Java and Kotlin according to 3 criterias: easy, support and performance and I gave each one a grade on 10. I chose some simple parts of a friend's app and is still in progress which is based on java and I converted those parts to Kotlin code and tested it out.
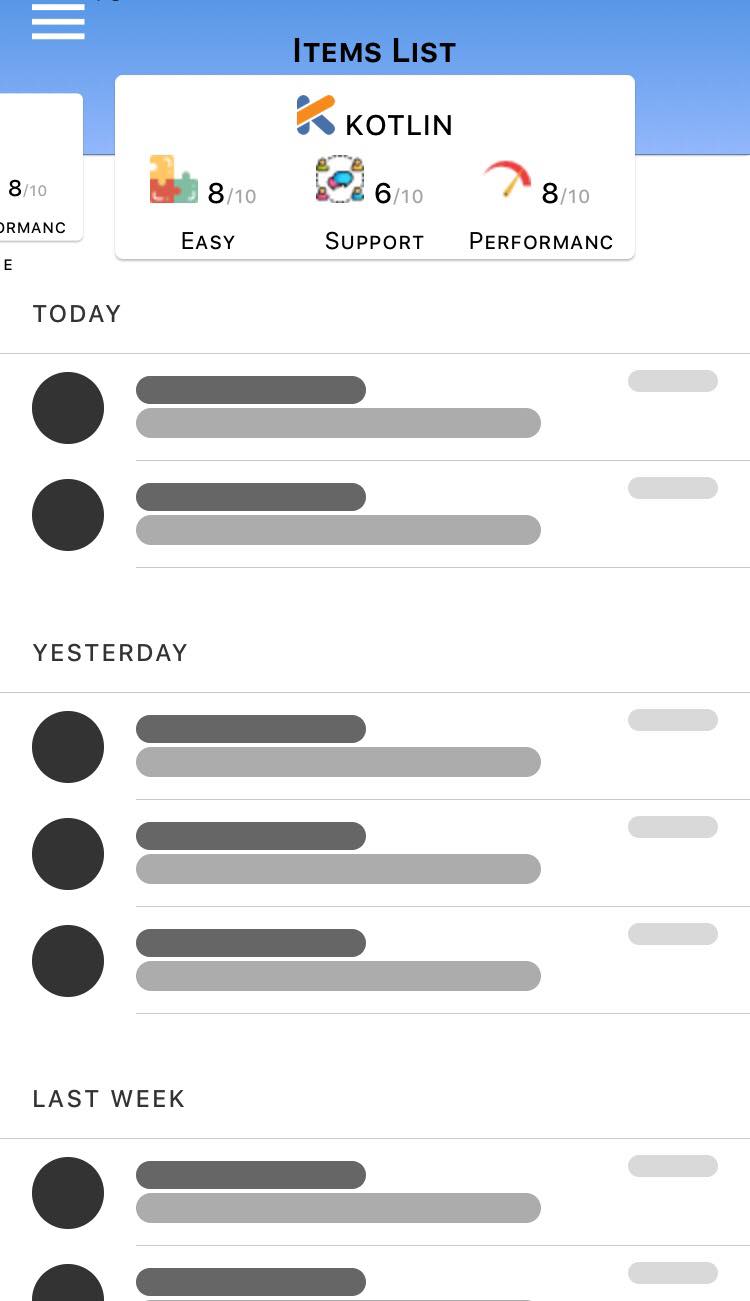
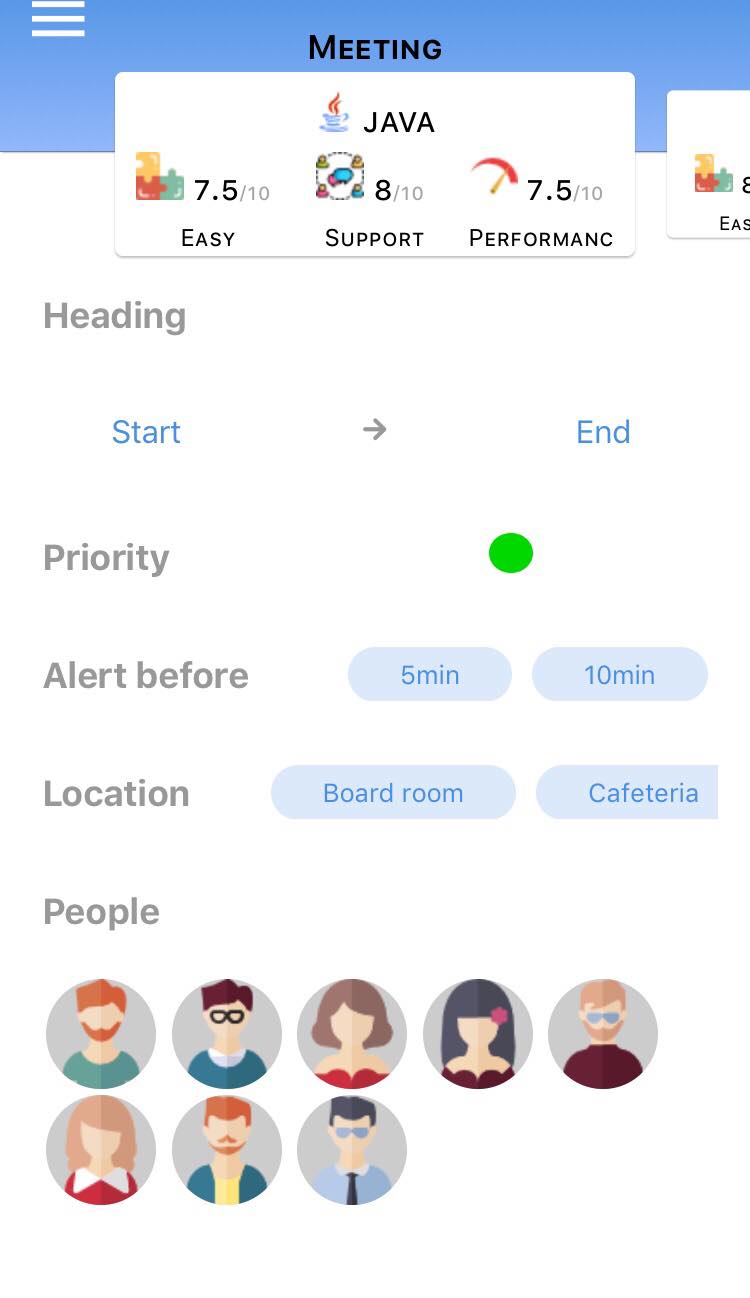
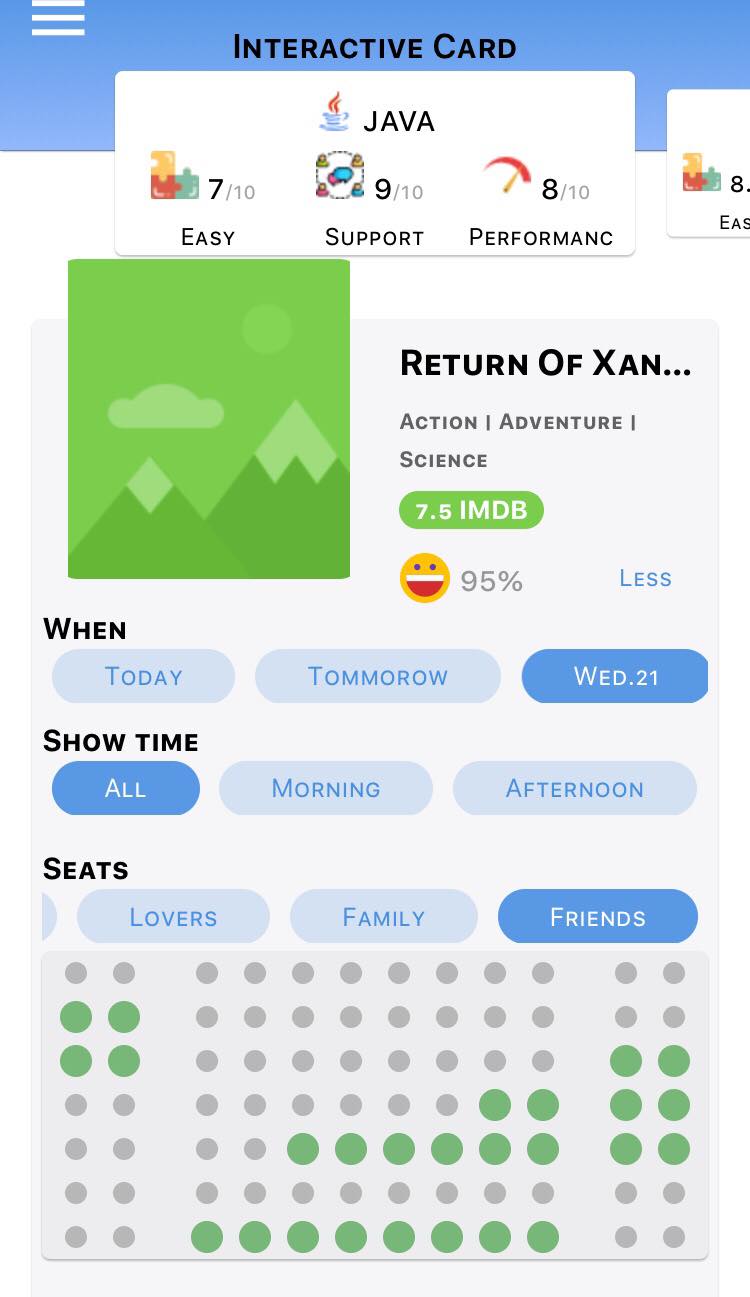
Java
Use case | Easy | Support | Performance |
---|---|---|---|
Item List | 7/10 | 9/10 | 9/10 |
Interactive card | 7/10 | 9/10 | 8/10 |
Meeting schedular | 7/10 | 9/10 | 8/10 |
Kotlin
Use case | Easy | Support | Performance |
---|---|---|---|
Item List | 8,5/10 | 8/10 | 7/10 |
Interactive card | 8,5/10 | 8/10 | 6/10 |
Meeting schedular | 8,5/10 | 8/10 | 6/10 |
Conclusion
We notice that in these use cases code compiles slower while using kotlin but it becomes faster with incremental build so I decided to keep the results of the first compilation attempt. When it comes to code size, Kotlin requires less code than Java so that's why I increased the Kotlin's score on the 'Easy' column. And lastly, when it comes to the developers community of both languages, I gave a slighlty lesser score to Kotlin because its community is still sparse compared to Java. Therefore, documentation, tutorials or information about Kotlin are still not handy.
Paradigms
Object oriented programming
OOP is based on three main concepts: encapsulation, inheritance and polymorphism while the main character is the object. It belongs to the imperative family.
But what is the innovation of object oriented programming?
Here's an example of a plain java class:
Public Class Person{
private String name;
private int age;
private String function;
private Person(name, age, function){
this.name = name;
this.age = age;
this.function = function;
}
public void eat(){
//TODO
}
public void sleep(){
//TODO
}
public void work(){
//TODO
}
}
We call name, age and function class attributes and they represent our data and eat, sleep, work are functionalities. Those functionalities are the motor of our data, so Object Oriented Programming aims to gather these two elements in one single entity called a Class.
Still isn't that clear? Just hold on.A class is some sort of a prototype or a template of an object, an object with certain caracteristics that describes it; We can imagine that this object is you or me, and those caracteristics are your name or your age those are data (attributes) and also you as a human being, you do some actions like eat, sleep, drink or dance, thise are functionalities (functions or methods). Anyways, the "you" here is what we call an Object.
Functional programming
Functional programming is based on composing pure functions, avoiding shared state, mutable data, and side-effects, it belongs to the declarative family
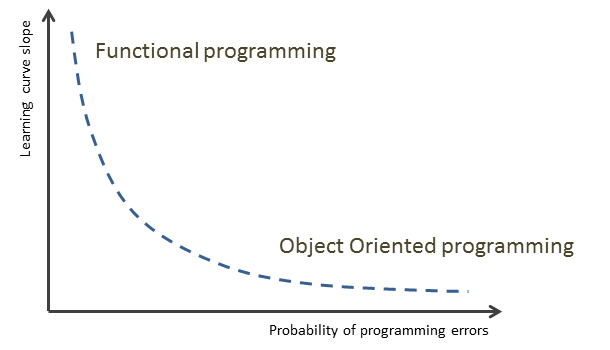
Src:https://dzone.com/articles/object-oriented-programming-strikes-back
Functional programming had its roots from mathematical concepts. It considers that all computations is the execution of a series of mathematical functions. So functions in Functional programming have some restrictions that functions of other programming languages don't have, the basic restriction is that these functions can't change their inputs also once the variable is declared, it can't be changed.
This paradigm focuses on computing resulats rather than performing actions, here is an example of a code using the imperative approach:
const triple = (arr) => {
let results = []
for(let i = 0; i < arr.length;i++){
results.push(arr[i]*3)
}
return results
}
const sum = (arr) => {
let results = []
for(let i = 0; i < arr.length;i++){
results += arr[i]
}
return results
}
Now here's the same code in the declarative style of functional programming:
const triple = (arr) => arr.map((current)=> currentItem*3)
const sum = (arr) => arr.reduce((prev,current)=>prev+current,0)
Both codes above give the same results but the code of the functional programming is shorter and more elegant. In general, functional programming isn't this simple and it takes a bit more time to learn. One of the languages that are adopts pure functional programming paradigms is Haskell.
How functional programming is used currently?
Python, Scala and Javascript, these are examples of funtional languages. It is used based on the domain of application. For instance, Scala is used in the Big Data Analytics field as Apache Spark is the most popular distributed data processing framework, and Spark is written in Scala. For machine and deep learning, Python is the most used and Javascript is used to master the frond-end functionalities.
In our case, Java has always been an object oriented programming language until Java 8 and it started slightly to borrow some Functional programming styles with lambda expressions for instance.
But the problem is that android support only Java 7 so that's why Kotlin creators decided to bring more Functional programming into Kotlin which makes it an Object Oriented language with a bit of a Functional programming style.
A side note about lambda expressions for those who are not familiar with this concept. Let's start with an example of a simple lambda expression:
listOfDrinks.stream()
.filter(arg -> arg.getDrink() == WATER)
.collect(Collectors.toList());
The stream function creates a stream out of our collection "listOfDrinks" and then its filtered such as each element of the list is represented by an argument 'arg' and finally we collect the results in a list again, this terminal action is necessary in lambda expressions.
And no, abusing of the use of lambda expressions does not mean that it is a Functional programming, it would more like respecting the immutability of the state for instance everytime a function is called, the same input must return the same output. So does that make Kotlin a Functional programming language?
Definitely not.
it still lacks some of the most important features like Higher Kinded Types and Typeclasses but the goal isn't to switch to FP but rather just take advantage of some of its useful features like inline functions.
Critics
The question to be asked now is: what is the best way to benchmark programming languages?
Actually, we can't benchmark programming languages, we can only benchmark programming language implementations. For example there are big differences between the performance characteristics of Ruby MRI and JRuby, or CPython, PyPy and IronPython.
Besides, all language implementations use compilation and virtual machines (most of them); it's just that in some the virtual machine is a temporary abstraction that is weaved in the code and disappears, while others keep it around during execution.
Anyways, the best way to benchmark them is to write the complete, real, working, application in both languages, and then measure parameters we are interested in. For instance, code size, time it took to develop, maintainability, processor usage, memory usage, latency ...
The hard part is making the benchmark actually represent the language implementation's performance, and not our relative skill level at the different languages and environments.This is the limitation of this approach that I've followed is that it doesn't quantify the fact that i'm a total beginner in Kotlin's programming language that I might have skipped some amazing optimization details while building the test applications test.
The worst way that is still not completely insane is the benchmarks game here Computer Language Benchmarks Game. They have a set of synthetic tests that stress different parts of language implementations, which we can probably use to make high-level predictions about how your actual program is about to behave. The reality will be different because these systems (the software and hardware) are so complex that nobody can make reliable predictions so it's easier to just write the program and profile than try to accurately predict how it will perform.
Eventually, this approach has its limitations as it doesnt measure performance using a real application (although I used a test application but still basic). In other words, attempts at running programs that are much simpler than a real application could lead to performance pitfalls. Also not all performance aspects were tackled during this benchmark as I focused on three aspects only: Language features, build time and programmation paradigms. The computer programs used for compiling this banchmark data in this section may not have been fully optimized, the most accurate benchmarks are those that are customized to a particular situation. So it's better to avoid over-generalization.
Conclusion
I guess after invoking these different aspects and based on our specific though limited benchmark, we can now say that Kotlin is probably the best for Android applications as it seems more concise and progressive and for a language that just started it has quite plenty of ressources on the internet and an ethusiastic community. Neverthless, I still think for a good programming grasp of concepts, it is likable to learn and be comfortable with Java before moving to Kotlin.
Credits
I was inspired by these sources: